Customize Chart.js Tooltip
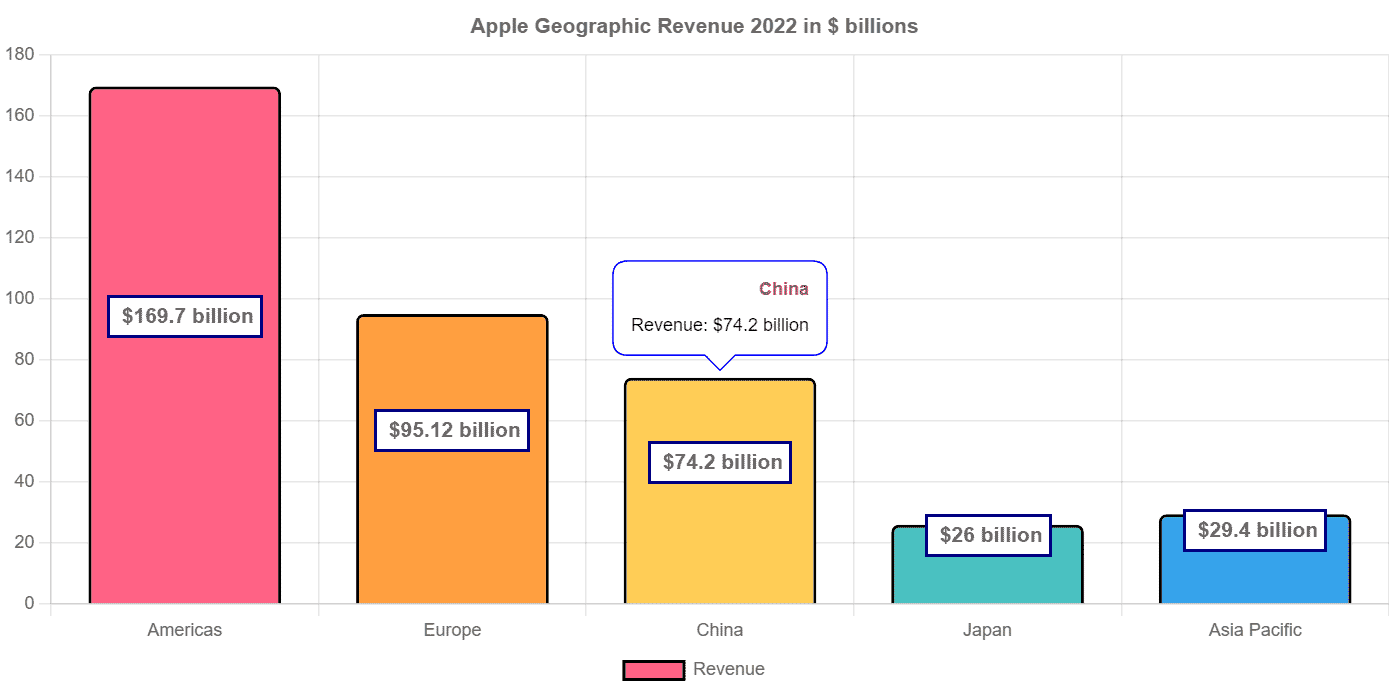
How to Customize the Tooltip in a Chart Drawn with Chart.Js
Chart.js is an excellent tool for drawing and presenting charts on your website. It is the most popular javascript charting library based on the number of npm downloads and github stars. You can use this charting library to draw various types of charts including line chart, bar chart, mixed charts, area chart, pie chart, doughnut charts and so on.
The best thing about chart.js is that compared to most other javascript charting libraries, it is comparatively simpler to use and to draw the basic types of charts you do not need to be a master of js. Moreover, this charting library offers excellent customization options so that you can draw and present highly attractive charts even with huge datasets.
In this tutorial, we will show you how to configure the tooltip in chart.js. A tooltip is the small box type element that appears when you hover over a chart. Generally, the tooltip includes the label and the value.
You can further customize the tooltip to change its appearance and the data shown in the tooltip. The small arrow appearing at the bottom of the tooltip generally is called caret and can also be customized. If you want, you can change the background color of a tooltip, text size, color and so on.
The configurations for tooltip can be set up inside plugins. Options.plugins.tooltip
The tooltip can be enabled and disabled depending on your need by setting enabled to true or false. By default, it is set to true and to disable it, you will need to set it to false. The default color of the tooltip is black but you can configure it and change it according to your need.
Here are the main configurations to be used with tooltip:
Enabled: Takes the value true or false and allows you to enable or disable the tooltip.
External: allows you to set and use a custom html tooltip.
Mode: Several interaction modes are available including point, nearest, index, dataset, x and y. Intersect: can be true or false and when true, the tooltip mode is applied only when the mouse intersects an element, otherwise, it is active at all times.
Position: Defines the mode for positioning the tooltip. Two possible modes are average and nearest but you can also define your own custom modes.
Callbacks: There are many callbacks available for tooltips that can be used to change the tooltip content, for example, add a $ sign before the values.
ItemSort: This function allows for the shorting of the tooltip items.
Filter: This function allows for the filtering of tooltip items.
backgroundColor: Allows for changing the background color of the tooltip.
titleColor: Allows to set the color of the title.
titleFont: Allows to change font settings for the title including font size and font weight.
titleAlign: Used to horizontally align the title text such as ‘left’ or ‘right’.
titleSpacing: Spacing added to the top and bottom of the title line.
titleMarginBottom: Margin to add on the bottom of the title of tooltip
bodyColor: allows to change the color of the body text in the tooltip.
bodyfont Allows to change the font settings for the tooltip body text including font size and weight.
bodyAlign: Allows to horizontally align the body text inside the tooltip.
bodySpacing: Spacing to add to the top and bottom of each tooltip item.
footerColor: Allows to change the color of the footer text inside the tooltip.
footerFont: Used to set the font size and weight of the footer text.
footerAlign: Used to horizontally align the footer font.
footerSpacing: Spacing to add to the top and bottom of each footer align
footerMarginTop: Margin to add before drawing the footer inside the tooltip.
padding: The padding inside the tooltip.
caretPadding: Extend the tooltip arrow by moving its tip extra distance from the tooltip point.
caretSize: Size of the tooltip arrow in pixels.
cornerRadius: Radius of the tooltip corner curves.
usePointStyle: Use a different point style than the square color boxes inside the tooltip such as triangle, circle or star.
rtl: if set to true, renders the tooltip from right to left.
xAlign: Position of the tooltip caret in the x direction.
yAlign: Position of the tooltip caret in the y direction.
Now, let’s draw a simple bar chart and customize the tooltip. In case you want to know about how to install chart.js, you can do so easily by adding a cdn link to your website header. Here is the complete script you can use to install version 4.4.2.
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/chart.umd.min.js"></script>
Otherwise, you can also install chart.js using npm:
npm install chart.js
It will install the latest version to your server.
Now, here is the script to draw a bar chart and customize tooltip. We will first draw a canvas for the chart and then add the rest of the script to create the chart:
<div>
<canvas id="myChart"></canvas>
</div>
<script src="https://cdn.jsdelivr.net/npm/chart.js"></script>
<script src="https://cdn.jsdelivr.net/npm/[email protected]/dist/chartjs-plugin-datalabels.min.js"></script>
<script>
Chart.register(ChartDataLabels);
var ctx = document.getElementById('myChart');
new Chart(ctx, {
type: 'bar',
data: {
labels: ['Americas', 'Europe', 'China', 'Japan', 'Asia Pacific'],
datasets: [{
label: 'Revenue',
data: [169.7, 95.12, 74.2, 26, 29.4],
backgroundColor: [
'rgb(255, 99, 132)',
'rgb(255, 159, 64)',
'rgb(255, 205, 86)',
'rgb(75, 192, 192)',
'rgb(54, 162, 235)',
], hoverOffset: 70,
borderWidth: 2,
borderColor: 'black',
borderRadius: 5,
}]
},
options:
{ interactions: {mode: 'index'}, responsive: 'true',
plugins: { tooltip:
{position:'average', intersect: false, backgroundColor: 'white', titleColor: 'brown', bodyColor: 'black', borderColor:'blue', borderWidth:1, displayColors: false, rtl:'true', yAlign: 'bottom', caretPadding: 5, padding:12, titleMarginBottom: 10, callbacks: {
label: function (context) {
return context.dataset.label + ': ' + '$' + context.formattedValue + ' billion';
}
}, },legend: {position: 'bottom'}, title: {display: true, text: 'Apple Geographic Revenue 2022 in $ billions', font: {size: 14}}, datalabels: {align: 'end', Color: 'black', backgroundColor: 'white', borderColor:'navy', borderWidth:2,anchor: 'middle', font: {size: 14, weight: 'bold'}, formatter: function(value) {
return ' $' + value + ' billion';
},}},
scales: {
y: [{
beginAtZero: true,
}
]
}
}
});
</script>
The customizations made to the tooltip in the above script also includes a callback to include a currency sign in the tooltip value.
plugins: { tooltip:
{position:'average', intersect: false, backgroundColor: 'white', titleColor: 'brown', bodyColor: 'black', borderColor:'blue', borderWidth:1, displayColors: false, rtl:'true', yAlign: 'bottom', caretPadding: 5, padding:12, titleMarginBottom: 10, callbacks: {
label: function (context) {
return context.dataset.label + ': ' + '$' + context.formattedValue + ' billion';
}
}, },
It is a simple bar chart that shows the Apple company’s revenue from each major geographic region for 2022. While the default background color of the tooltip is black, we have changed it to white and also the colors for title and body fonts. To make the tooltip clearly distinguishable, we added a 1 px border. To make the corners more rounded, we can increase the cornerRadius for the tooltip.
The default value for cornerRadius is 6 which means 6 pixels and you can increase or decrease it according to your choice. If you want to increase the size of the arrow at the bottom of the tooltip, you can do it using caretSize. The default caretSize is 5 and increasing it will widen the arrow’s base and increase its size. With rtl set to true, you can see that the tooltip title is aligned to the right instead of being positioned on the left or in the center.